Introduction
This article will show, step-by-step with Descope Flows, how to configure advanced custom claims to support Hasura within you're application. This guide will also show you how to include these custom claims with our Node.js SDK.
What is Hasura
Hasura is a middleware service that exposes your database and back-end services as a GraphQL API. Using JWT with Hasura means adding the appropriate claims to your user's session JWT, which will determine their level of access. Adding these claims is easily accomplished by utilizing advanced custom claims within Descope.
You can find an example of the format of Hasura JWTs on their website.
Implementing Custom Claims via Descope Flows
Add the Custom Claims action
To add advanced custom claims within your Descope flow, you can open the applicable flow to which you want to add the custom claims. Once you have opened the flow, click the add button on the bottom left, search for the action "Custom Claims," and add it to your flow.
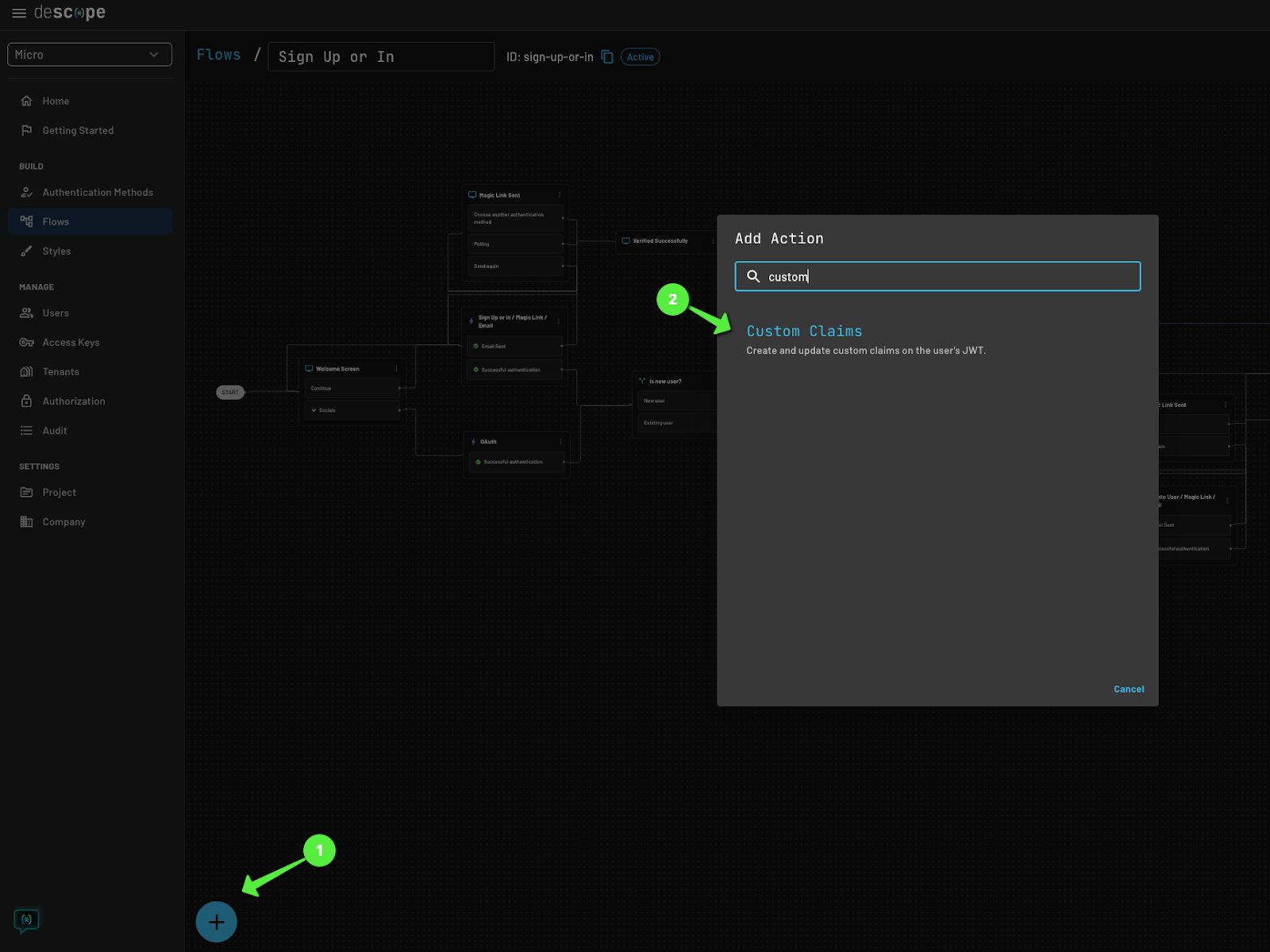
Switch to Advanced Configuration Mode
Once you've added the custom claims to your flow, select the advanced option within the modal.
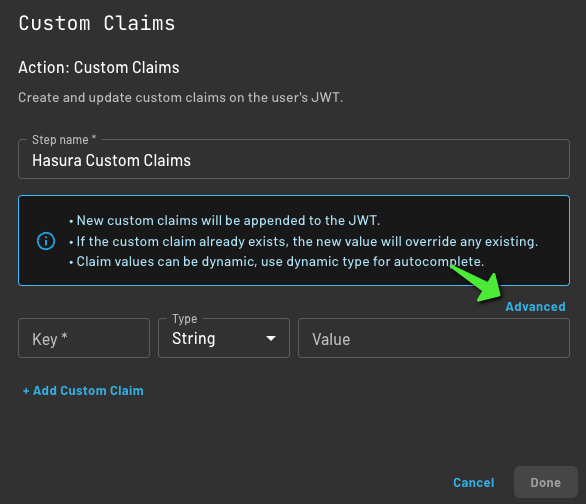
Customize the Action With JSON Formatted Claims
Within the advanced pane, you can add JSON including the custom claim you wish to build. You can also utilize Descope's dynamic values to input items like the user's ID.
For this example with Hasura, we will be adding the following:
{
"https://hasura.io/jwt/claims": {
"x-hasura-default-role": "user-role",
"x-hasura-allowed-roles": [
"user-role"
],
"x-hasura-user-id": "{{user.userID}}",
"x-hasura-org-id": "456",
"x-hasura-custom": "custom-value"
}
}
Once added, your action will now look like the following:
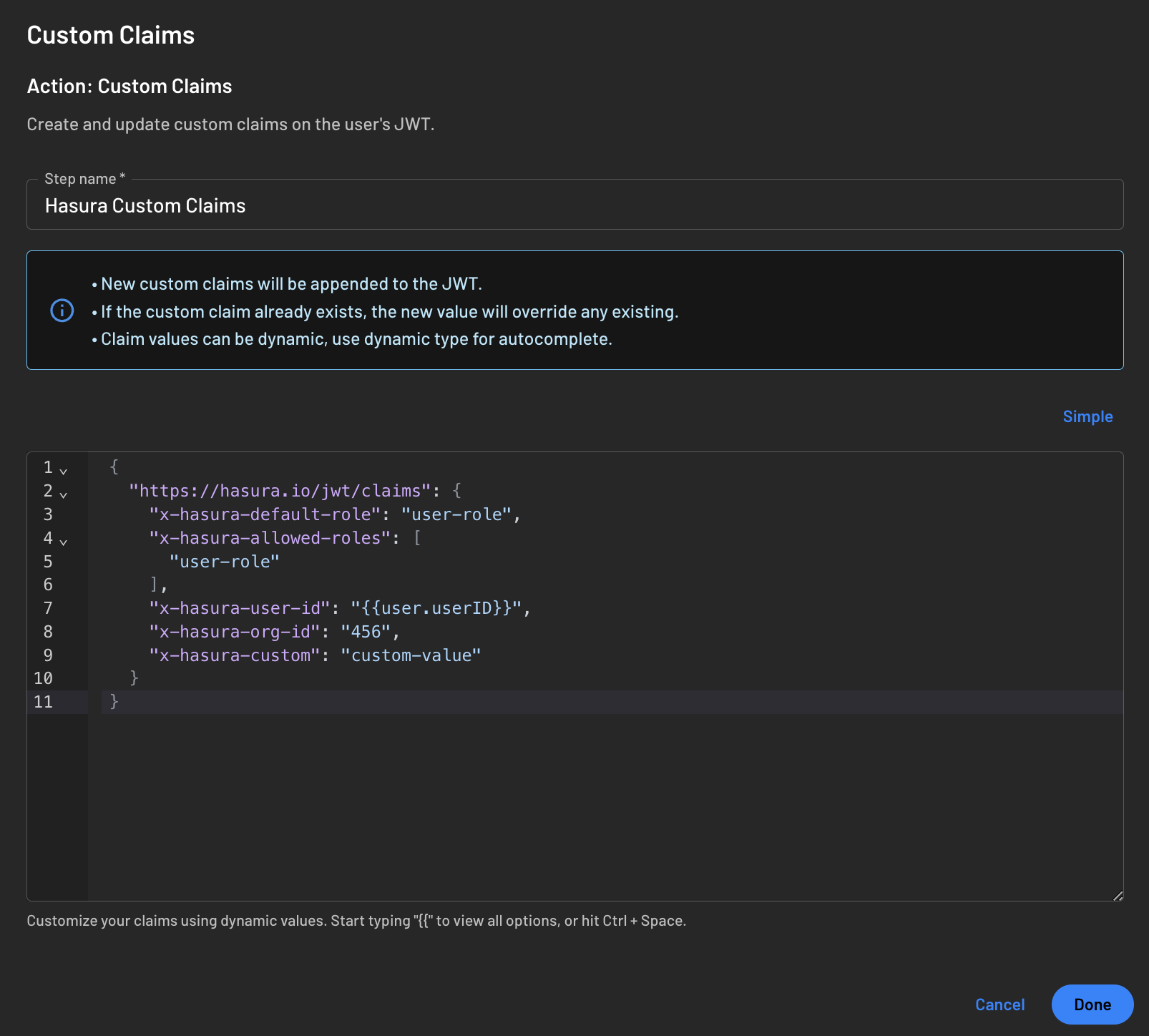
Save and Attach the Action
Click done on the action and then add it after the user's been verified. In this example, we will add it just before the end of the flow.
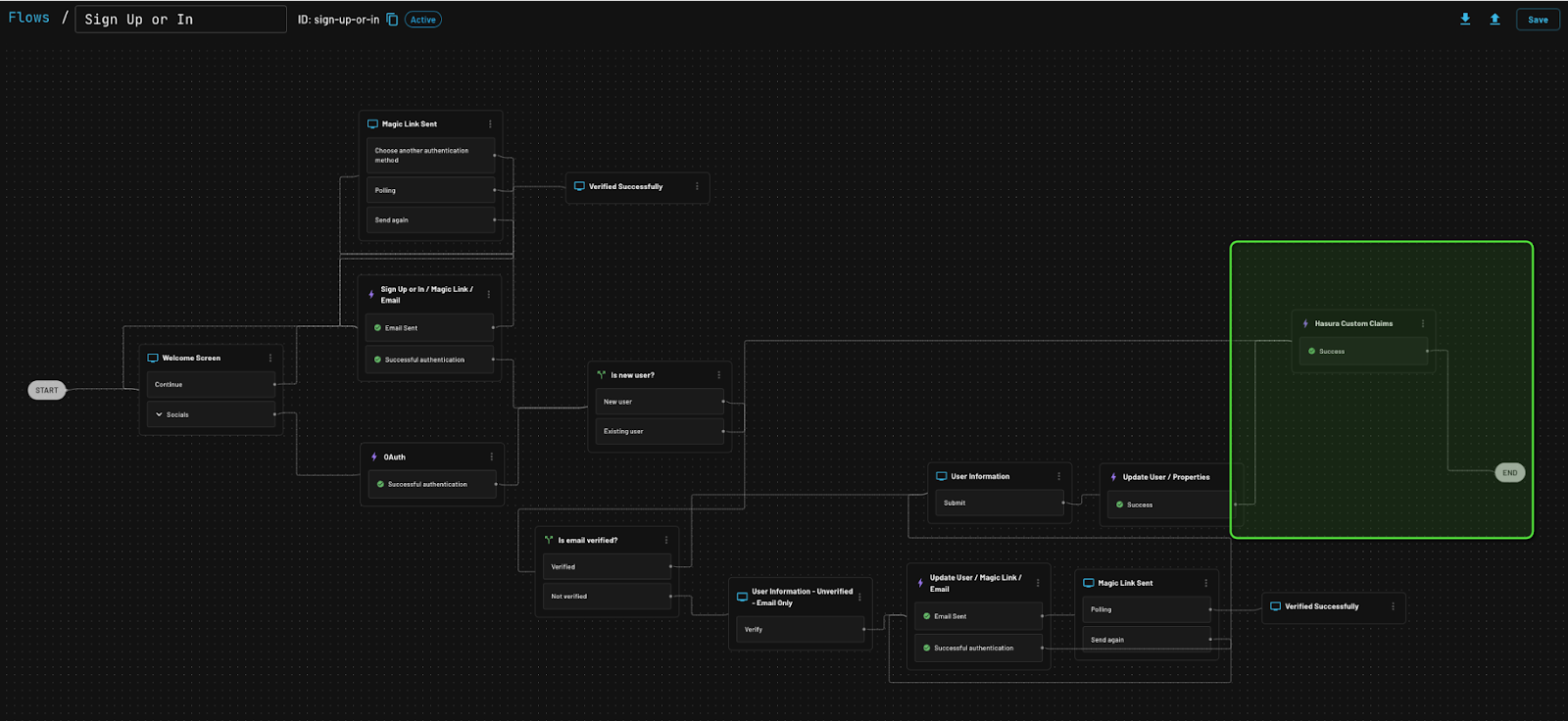
Test the Descope Flow
We can then test it within the sample app on the getting started screen.
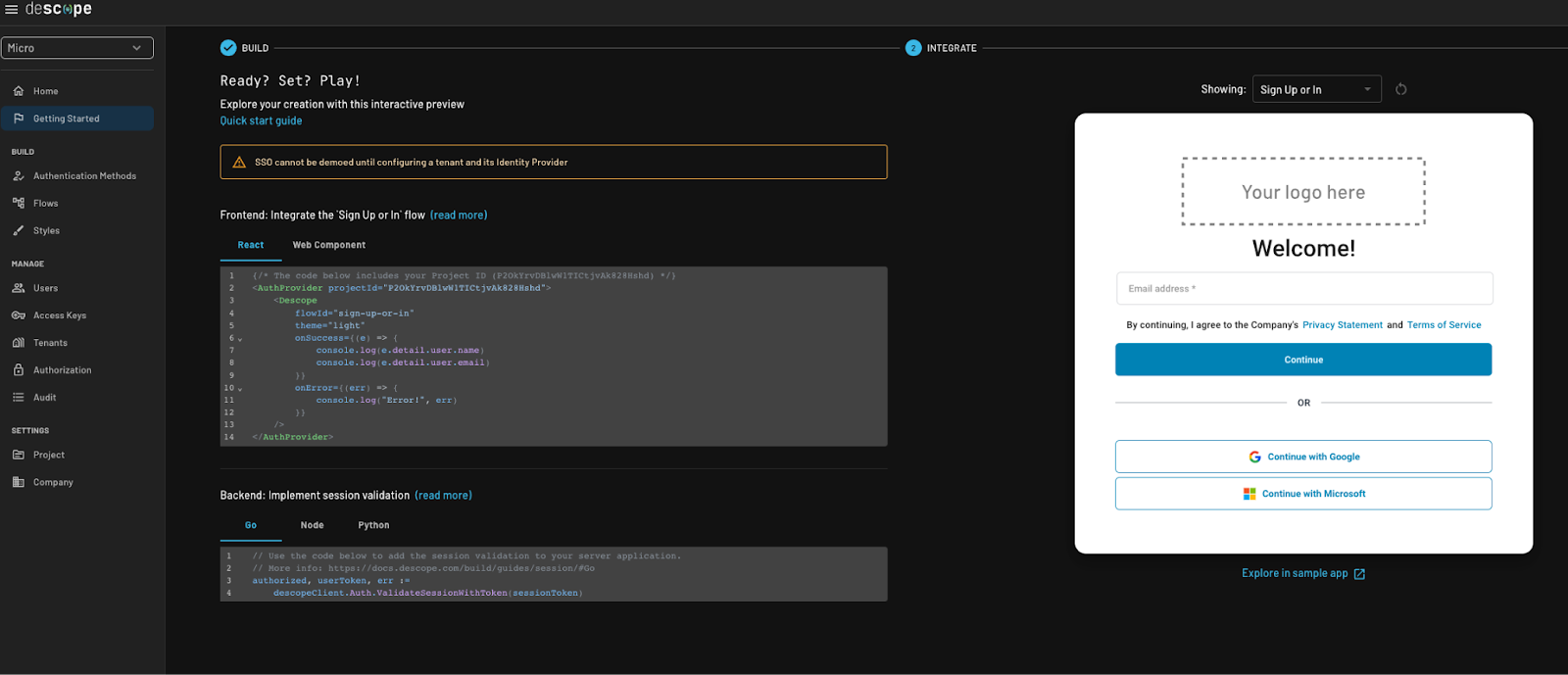
The advanced custom claims configured are now added to the user's session JWT.
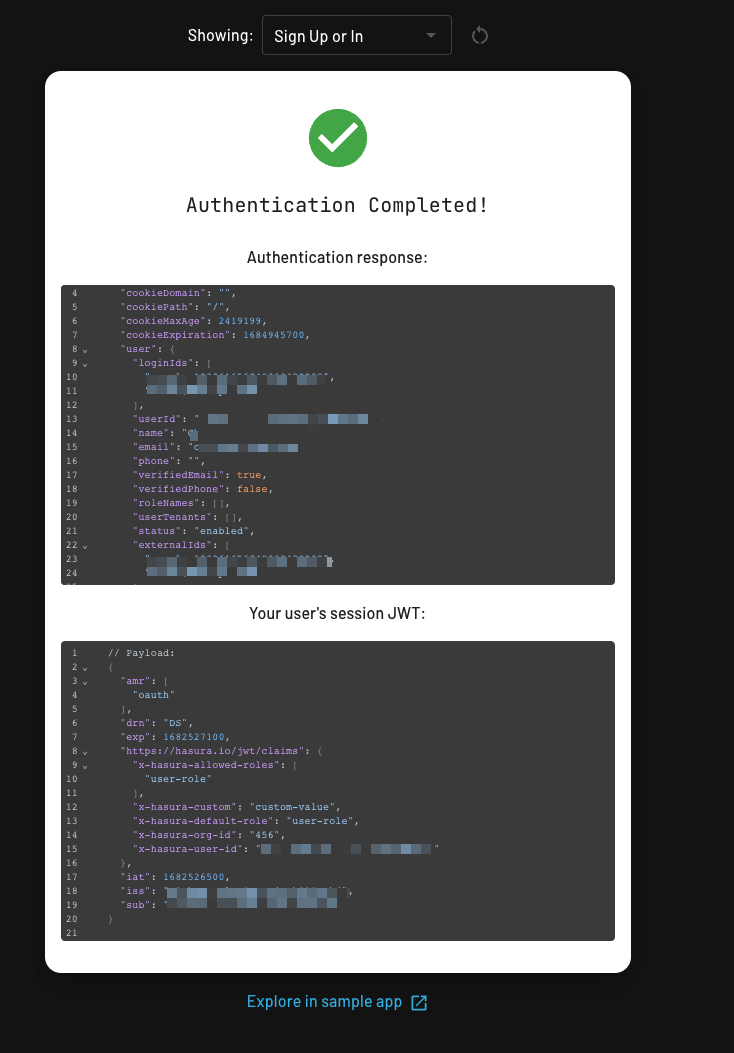
Implementing Custom Claims via Descope SDK
We will utilize the NodeJS update custom claims function. Details can be found here.
import DescopeClient from '@descope/node-sdk';
const client = DescopeClient({ projectId: '__ProjectID__', managementKey: "Your-Management-Key" });
// Args:
// jwt: the current/original session jwt of the User
const jwt = "xxxx"
// customClaims (dict): Custom claims to add to JWT, system claims will be filtered out
const customClaims = {
"https://hasura.io/jwt/claims": {
"x-hasura-default-role": "user-role",
"x-hasura-allowed-roles": [
"user-role"
],
"x-hasura-user-id": "xxxx",
"x-hasura-org-id": "456",
"x-hasura-custom": "custom-value"
}
}
const resp = await client.management.jwt.update(accessTokenStr, customClaims)
if (!resp.ok) {
throw new Error('Error processing custom claims')
}
else {
console.log("Successfully updated jwt.")
console.log(resp.data.jwt)
}
The returned user session JWT will have the Hasura information as expected:
{
"amr": [
"email"
],
"drn": "DSR",
"exp": 1683040662,
"https://hasura.io/jwt/claims": {
"x-hasura-allowed-roles": [
"user-role"
],
"x-hasura-custom": "custom-value",
"x-hasura-default-role": "user-role",
"x-hasura-org-id": "456",
"x-hasura-user-id": "xxxx"
},
"iat": 1682526921,
"iss": "xxxx",
"roles": [
"TestyTester"
],
"sub": "xxxx"
}